So I’d like you to look at this image just for a moment. I’ll just let you watch it happen.
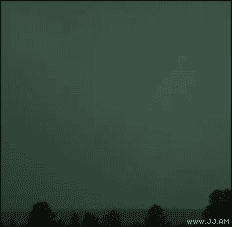
What it is, clearly, is lightning. And what you see happen is, you get a bang at the top left and then it starts forking, and each fork forks again and forks again, until finally one hits the ground and all the others die away, and then you see the electrons transferring back up to the cloud. So I’ll just let you watch it once more and then say why I’ve used this.
Funnily enough, this is very like a certain style of programming called “backtracking.” It’s an amazingly powerful programming method. Imagine that you’ve got to get yourself through a forest by following a path, and you don’t know all the different turns which way to go. The way to solve it is by using backtracking. Whenever you come to a fork in the path, you take one of them, and if that doesn’t get you where you want to go then you backtrack to that point and then you try the other. If that doesn’t work, come back and try another. That works all the way down.
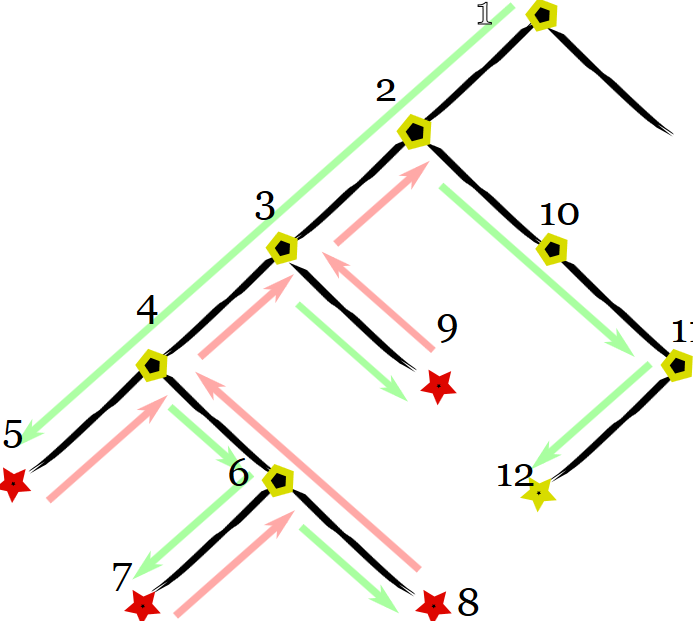
This is an example of the sort of tree that you get while you’re trying to do this with computers. At each decision point you’ve got a state, and at each decision you have to try one of the options, and you keep trying until it finally fails, like at point 5, and then you backtrack one point and then you try that way, so then you go to 6 where you’ve got to make a decision again. You go to 7, it fails, and then back. And you keep backtracking until you find a route through that succeeds.
There are several different ways of doing this when you program it. You can do it serially, as I’ve just described, where at each point you just try one of them and then go back. Or you can do it in parallel, where at each point you split off more programs to do all the options simultaneously. You can also just do it just to find one solution, which might not be the best solution but at least you’ve got a solution. Or you can try it to find all the solutions and then decide which was the best.
And the last thought is heuristic, where there are so many options at each point that you couldn’t possibly do them all. So then you have to do something smart at the decision point to decide which sub-set of the decisions you’re going to take.
HOW TO RETURN (route, state) path.to aim:
IF state = aim:
RETURN ("success", route with state)
IF state in route OR deadend state:
RETURN ("failure", {})
PUT route with state IN new.route
FOR option IN options state:
PUT (new.route, state altered.for option) path.to aim IN result, route
IF result = "success":
RETURN ("success", route)
RETURN ("failure", {})
I’m not going to explain this program to you, but this is the program that just does all that. It’s a surprisingly small amount of code that solves an amazingly large class of problems. I wrote an article about this and I used it to solve a lot of problems just to show that this one unchanging program could solve so many problems.
So for instance the Farmer and the Produce Problem. That’s where a farmer has got a bale of hay and a goat and a dog, and has to cross the river in the boat but the boat will only hold two of those things. So he could only get two in the boat and then go to the other side and then come back to get the other. But he must never leave the goat with the hay, or the dog with the goat. So that’s the problem and you’ve got to solve it. This program will solve that.
The Two Jugs Problem. You’ve got two jugs of different amounts, let’s say five liters and three liters, except the recipe says you’ve got to measure out one liter. How do you do that? Well, one of the solutions is you fill the three-liter jug up, you pour it into the five-liter jug, you fill it up again, you pour it into the five-liter until it’s full, and then you’ve got a liter left. This program will solve that.
It will solve the Towers of Hanoi Problem where you’ve got a tower of discs, each of smaller size, and you have to move them from one peg to another. You’ve only got three pegs; you’ve got to move them from one to another. But you can only move them one disc at a time, and you must never put a large disc on a small disc. This will solve that. In fact, when I tried it out just for two discs it found twelve solutions, and I hadn’t realized that that was possible.
But it will solve the Eight Queens Problem, where you’ve got to put eight queens onto a chessboard so that that they can’t take each other. It’ll solve Sudoku, and many many others.
By the way, this program is written in ABC, and ABC was the programming language that Python was based on. The reason was because Guido van Rossum and I used to work together.
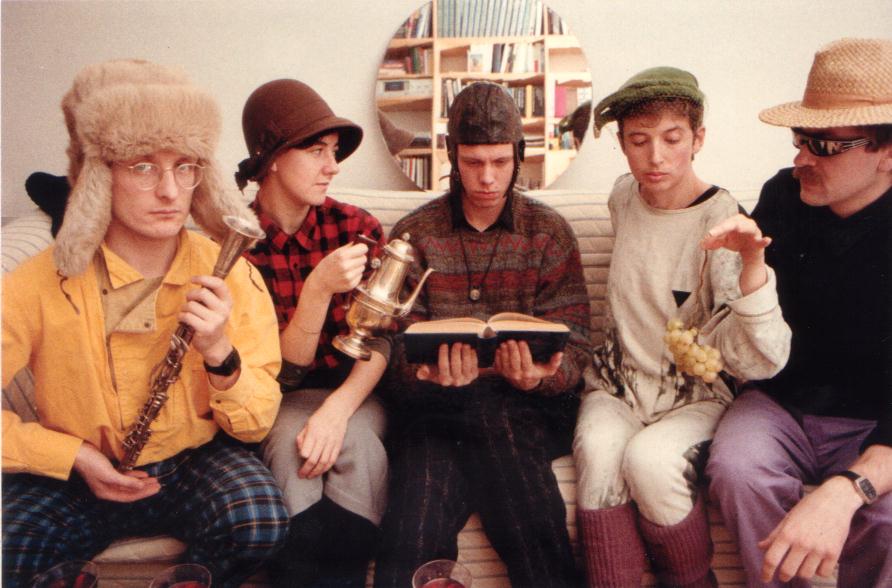
That’s me in those days. That’s Guido in the middle. This was at a party. They tell me it was a very good party, but I don’t remember anything about it.
The funny thing is, evolution works very similarly to how backtracking works, and now that I’ve told you the words, it’s heuristic, and that is because each state of the program as it were is a person, you or me or a plant or an animal. And you could produce millions of possible children, but you don’t. You just produce a small number. So that’s why it’s heuristic. It’s parallel because we’re all surviving at the same time and we’re all doing this similarly. It’s got a similar definition of failure, although it does have a different definition of success.
There’s this great Dutch book called Cheese and the Theory of Evolution, unfortunately not translated into English. I might do it one day if I get the urge to. It’s a great book. But anyway, it explains that you only need three things for evolution to work. You need variation, you need replication with inheritance, and you need competition. Let me treat those one by one.
So, variation. The creatures that are evolving need to be different from each other in order to be different so that they can compete. In the early days of evolution, the only source of variation was mutation. And mutation is actually an extremely bad way of generating variation because it’s almost always leading to deleterious effects. It’s like taking a recipe and let’s just take one ingredient at random and change it in some way. So, “I know, let’s make this cake with, instead of sugar, salt, and try it out. Oh no, that’s disgusting. Okay, maybe we didn’t put enough salt in. No, that doesn’t work either.”
So it’s a pretty bad way of doing it. But luckily, evolution had a long long time to work over, and so occasionally an advantageous mutation occurred, and so things did slowly get better.
However, once sex evolved, which of course took a long time, all of a sudden there was a huge source of variation. Because now you’re combining two lots of genes to create new creatures and that combination created a whole new pool or variation. And furthermore, they were combinations of two things that had already been very successful. So this explains the success of sex, by which I mean why such a large number of organisms use sex for procreation, plants as well as animals.
Now, competition. You’ve got to have competition because if everything survived then there’d be no selection of advantageous genes. We’re guaranteed eventually there’s going to be competition because if everything survived, they’d get bigger and bigger and more and more of them, and in the long run there won’t be enough resources to keep them alive. So we are ensured that there’s going to be competition.
And death is evolution’s backtracking. That is to say that’s the point of failure. And as I’m going to explain more later, death without offspring is actually the failure. So if you’ve had offspring and you die, that’s alright. You’ve succeeded. But the definition of offspring has changed for humans, as I’m going to be demonstrating in this talk.
The environment affects the definition of success, actually. And the environment changes and you have to adapt to the new environment, and therefore it redefines success. This is how species happen. A species, as we heard earlier this week, is a group of animals that can procreate amongst themselves but not with others. So the definition of species is whether things can procreate with each other.
It’s quite interesting to think that if you go back in evolution, there was some time in the past where cats and dogs meet up. That is to say there’s a common ancestor of both cats and dogs, and that the children of that ancestor of cats and dogs had the first cat and the first dog. And if you want to know what a dog-cat might look like, it’s interesting to know that a hyena is actually a cat and not a dog.
Camouflage is one of the results of the effects of the environment, because then that’s going to be advantageous for you because you’re not going to be so easily caught and eaten. But of course anti-camouflage is also advantageous for some animals like wasps. The reason that kingfishers are so easy to see is because I’m told they’re disgusting to eat. I’ve never tried it myself, but the reason that they’re so brightly-colored is to warn predators off from eating them because they are totally disgusting to eat.
Selection of advantageous genes [has] surprising side-effects. Here’s a very nice example. Belyaev in Russia bred foxes to try and breed tame foxes. So he only selected foxes for tameness. But it had the surprising effect of changing how they looked, so that his tame foxes didn’t look like foxes anymore. They looked just like regular dogs.
So the accretion of successful genes you can see as a form of memory. That it’s a very slow-forming memory, and it’s a read-only memory. You can’t change it in any way. But it is a memory of what’s been successful in the current environment in the past. In that program I showed you, there was a thing called the route, which saves the route that you’ve taken to get to the successful position so that you’ve got the answer to your problem. And you can see the genes as the equivalent of the route in that program.
Just to give you some examples of genetic learning as it’s happened in humans, why do we like sugar so much? Well, because one of the important things in the past for humans, before they could talk let’s say, so very very early humans before language evolved, and important thing was to eat enough. Starvation was one of the main forms of death. So how do you get the message to humans, how do the genes as it were, tell humans that something has got a lot of calories in it? Well, if it’s sweet, it’s got a lot of calories in it, so therefore somebody who evolves a gene to like sweetness is going to then eat more of those things and therefore are less likely to die of starvation. Similarly with fat, fat has lots of calories and calories were exactly the things that early humans needed. So the ones that developed a liking for fat were the ones that were more likely to survive. Of course that’s bad luck for us now, because now that we’ve got enough food, it’s working against us because we tend to eat too much sugar and too much fat. But that’s because it’s programmed in us genetically to eat these things.
Sex being fun is another example of something that we’ve learned. That is to say, if you’ve got a population of people who are neutral to sex and then a gene comes up that makes you enjoy sex, then that gene is going to get a lot of preference, of course, because the person who’s got that gene is going to be producing lots and lots more—well he’s going to be having more sex and therefore is more likely to have children. So therefore that gene is going to be advantageous for a sexual creature. So the reason why most of us find sex fun is because that gene has just spread right through the gene pool.
And age differences in couples you can explain genetically as well. It’s much more likely that you’ll see and older man with a younger woman than the other way round, a younger man with an older woman. And that’s because, if it’s genetically-programmed, then this gene is going to get far more favorably treated than the other way around.
So after sex developing in evolution, true memory was the next major development, because true memory, where you remember things yourself rather than just in your genes, allows you to learn behaviors. It allows to adjust your behavior to what’s happened to you in the past to recent outcomes to help you decide how to act.
The Sphex wasp is a good example of something that doesn’t have any memory. What a Sphex wasp does is it makes a hole in the ground to make its nest, it lays its eggs, it goes off and it gets a prey, a caterpillar or something which it then stings to paralyze it, drags it back to the nest, walks into the nest, checks that the nest is okay, drags the caterpillar in, and then seals up the nest and goes away. And of course when the baby wasps come out, they eat up the prey.
What they’ve discovered with the Sphex wasp is, at the moment when it drags [its prey] to the mouth of the nest and goes in to check, if you pull that prey away a few centimeters, when it comes out it says, “Hey, where’s my prey?” And then it finds it and pulls it back in again, and then it goes and checks again whether the inside of the nest is okay. And you can do this hundreds of times and it will continually check whether the nest is okay before dragging it in. So it has no memory whatsoever. It’s entirely instinct-based. So when you’ve got memory, all of a sudden you can base your decisions whether you’re going to survive or not on past experiences rather than just pure instinct based in your genes.
I’m going to talk about why we like certain things, and so I want to talk about awareness. Hypnosis is an example of where things happen and we’re not aware of the real reasons. What happens under hypnosis, if you’re told to do something, for instance “if you hear the world London, open the window,” when you come out of hypnosis and you’re sitting there working or whatever and then you hear the word “London” and you go and open the window and somebody says, “Why did you open the window?” They’ll say, “It was getting stuffy.” They won’t be aware of the real reason; they will rationalize after the fact.
There’s a great book, Surely You’re Joking, Mr. Feynman! by the Nobel prize-winning physicist, who underwent hypnosis just to try it out. He was even aware that he’d been told to do something, and he did it anyway. And he was saying, “All the time, I was saying to myself I could do that,” in other words do [a] different thing than he’d been told, “but I won’t.” And what he said is that’s just another way of saying you can’t. So in other words, very often while under hypnosis, you do things without knowing the real reason for it, and you rationalize a new reason. And there are some people who think in fact there’s no free will at all, that we always rationalize after the action. And there’s some evidence for that. I don’t know if I believe it yet, but at least there are people who believe that free will doesn’t exist and it’s always post hoc rationalization.
So we are in a profession that’s predicated on observing people and doing things to make them happy. So it’s fun, then, to watch people and watch out for things that people apparently love for some reason or another, because they cluster around it or something, and try to postulate what would be the evolutionary advantage that this represents. So let me give you some examples.
Water. Whenever there’s water, people love to cluster around it. I was driving through England with my children, and every time we passed a river or a lake or something, they’d say, “Stop! Stop! Stop!” and they’d be happy to play at the water’s edge for hours. Well, you could imagine evolutionary advantage for water. Water is an essential resource, and so if you ended up by water, if you just liked being there then you would stay there and then you would have a source of water. But if you didn’t like being there, then you might travel on further and then eventually die of thirst.
Heights is another thing that people cluster around. This is the Sacré-Cœur in Paris. Beautiful building right at the top of a hill. And of course any tourist who goes there goes to see the Sacré-Cœur. They go in for twenty minutes and look at the inside of the beautiful building, and they come outside and they sit on the steps outside for hours, just looking at the view. So clearly, height is something that people really love to do. They’re just happy to sit and stare for no reason at all. It just makes them feel good.
Fire is a similar sort of thing that people just like to sit around and just stare into the fire, and they’re willing to sit there for hours. And it’s interesting that humans are very different from most animals, because most animals flee from fire as fast as they can. You can understand the reason for that, because if there was ever a gene for animals in the forest, “Hey look, there’s a fire! Let’s go and watch it,” of course that gene would die out fairly quickly. So it’s surprising, then, that humans have this gene for liking fire.
And I have to tell you a story which made me think differently about why people like fire. Because there are obvious advantages to liking fire in the distant past. For instance of course it keeps you warm. But also since most animals are frightened of fire, then they won’t come near you at night, and it’ll keep you safe during the night while you’re asleep. So there are obvious evolutionary advantages.
But the interesting story is that a colleague of mine was babysitting for another colleague of mine who happens to be sitting in the audience. And this baby was crying and crying and crying. And he did not know what to do. In the end he strapped the child onto the baby carrier and went for a walk with her to try and calm her down. At the end of the street there was a vending machine, a cigarette machine or something, and on that vending machine there was just one single red LED light. The child saw this and it stopped the child from crying, and the child was just staring at this red light, completely transfixed just by this red light. And was happy, having cried for so long. And it made me think maybe the reason we like fire is because it flickers. Because an LED flickers. It flickers very fast so that we don’t see it flickers, but it does flicker. If you move your eyes quickly, then you can see that it’s flickering, because you see points rather than a continuous line. But an LED does flicker, so maybe we have a gene that makes us like things that flicker, and that therefore the reason we like fire is because it flickers, and we don’t like fire because it’s fire, it’s just the flickering.
And that also got me thinking, are there other things that flicker that people are willing to sit around in front of for hours regardless of the rubbish that’s on it? Yes, there are things. Maybe people stare at the television for so long just because it tweaks the result of this flicker gene that makes us happy. Which makes me wonder about the future of e-ink because my suspicion then is that if some people are offered the same product, one with e-ink which doesn’t flicker and one with an LCD screen which does, they’ll look at it and say, “I don’t know why, but I just prefer the LED version.” So I suspect that in the long run that e-ink may—I mean e-ink does have some advantages like long battery life. But that people in the end do prefer to look at a flickering screen. Which might actually also explain why people get their phones out so often. Because it just gives you a good feeling. We’re not aware of this, but just by looking at the phone, we get this fire/good feeling effect.
So the title of the talk has the word “phenotype” in. The phenotype is the visual manifestation of your genotype. Your genotype is the collection of genes that you hold, and the phenotype is what gets built with that. That could depend on the environment. For instance if you grow up in a place with little food, you’re going to be shorter and smaller than if you grow up in a place where there’s lots of food. So the phenotype includes your hair color, your size, your aggression, your strength. And for some creatures… For instance bird nests, they’re built as a result of the genes, they’re not learned behavior. So it’s part of the phenotype. Beaver dams similarly, spider webs; they’re build by the genes but they’re not actually part of your body.
The next part of development for humans was the development of language. Because the advantage of language, the evolutionary advantage of language, is that it allows evolution with planning, because you’ve got these memories that you can now pass on to your offspring or your friends. So if you now have had an experience, you can say to somebody, “Don’t do that. I tried it,” or, “Don’t do that, you’ll hurt yourself.” And so this creates within genetics what are called memes. We misuse the word on the Internet to mean pictures of cats. But memes are actually a specific piece of information in analogy to genes, a carrier of information that gets passed around which may or may not help you in survival.
So things get built from memes, like houses or umbrellas, or the idea of washing your hands to keep you healthy. And some of them work and some of them don’t. So a bad meme… A couple of hundred years ago there was this idea that if you were ill, somebody should drain off some of your blood to make you better. That was clearly a bad meme and I’m glad to say it’s clearly died.
What this means now is it’s not only important to pass on genes in evolution, but it’s just as important to pass on ideas. In other words, ideas are just as important as having babies. I don’t really have time to go into Englebart, but Dough Englebart talked a lot about augmenting the collective IQ, which is the same sort of idea. McLuhan also points out that all these things that we produce with wheels are just extensions of our body. That it’s part of our extended phenotype, in other words.
And in fact, what’s the role of a conference? To exchange ideas. In fact, it’s the memetic equivalent of having sex. Or, in fact, it’s more like an orgy. Because everybody is exchanging ideas all the time, as…well, I’ll just let you think that for yourself.
So the extended human phenotype uses memes rather than genes to extend our abilities. So, protection helmets when we’re riding on bicycles or motorbicycles. Our clothes to keep us warm. Raincoats to keep us dry. Houses to live in. Memory, we can extend our memory now with writing, but [also] with photography and video. We can repair things. I mean, glasses… We don’t have to wait for evolution to work out how to repair our bad eyesight. We can repair it ourselves now. Medicine, hospitals. We can cure deafness now, just by using memes. Our abilities are greater. We can travel much much faster. We can fly. We can travel over water and under water. In fact, there are not many things that we can’t do. And now we’re extending our senses. Telescopes, of course. But now we’ve got weather radar; we can know what the weather’s going to be like in the next couple of hours pretty accurately. We can see whether it’s going to rain or not. And we’re the last generation that will ever get lost because with sat-nav we now have this sort of sense in our body, and that’s our telephone, which whenever we don’t know where we are we just look it up.
Now, the computer is being used for so many of these things that I claim that we have to consider the computer as part of our extended phenotype. It’s just a part of a thing that has evolved with us using memes.
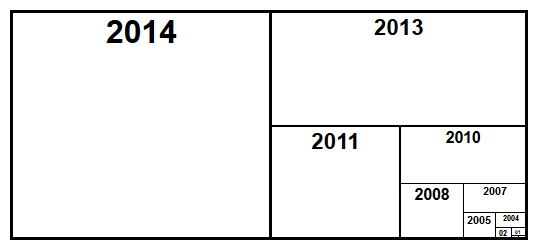
Moore’s Law has been going on for a long time, the power of computers essentially doubling every eighteen months. And a lot of people don’t really understand its true consequences, so let me just demonstrate what Moore’s Law has done for us. We take a piece of paper and we divide it in two and we write this year’s date on one side. That represents the power of your laptop this year. Now we’re going to take the other side and divide that in two and write the date eighteen months ago on the other half. That’s the relation ship of your computer now with one that you could’ve bought eighteen months ago at the same price. We’re going to keep doing that. Divide the rest of the space in half, and that’s the power of your laptop three years ago, so you can see that it was a quarter of what it would be now at the same price. And then we do that all the way back as far as we can go until our pencil runs out or we get bored or whatever.
What this shows you is that your current computer is more powerful than all the computers that you’ve ever owned, assuming you haven’t bought more computers than once every eighteen months, which I don’t know anybody who has. So in other words your current computer is more powerful than all the other computers you’ve ever had, put together.
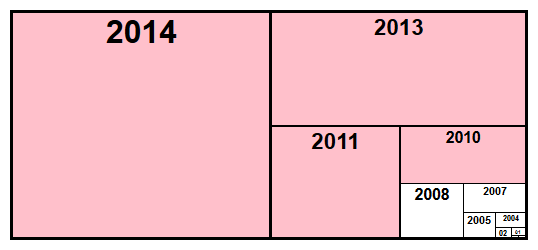
What’s also interesting is that if you look at this as a society’s level, computers last about four to five years, so if I know color red all the computers that are up to four or five years old, what you can see is that the amount of computing power in the whole world now is more than 90% of the computing power that has ever existed in total. So we have an amazing amount of computing power available to us.
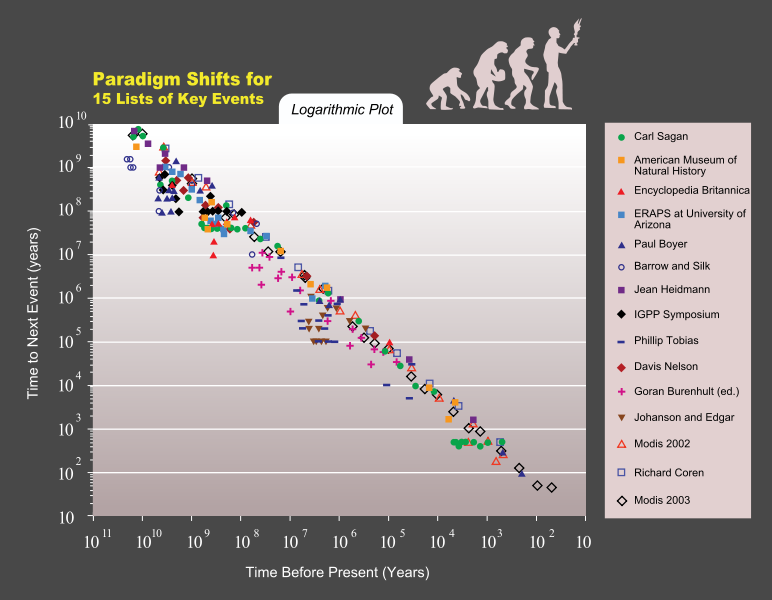
Image via Wikiepedia; graph originally by Ray Kurzweil
You may have heard of the Singularity. This is the idea of looking at how often paradigm changes happen. So we’re looking back to 10 to the 11th years in the past here. A paradigm change is when something essential changes that means you do things in a completely different way. The printing press was a paradigm change, but also the Iron Age was a paradigm change from the Stone Age. Digital money was a paradigm change. Trains, the steam engine was a paradigm change.
What the person who drew this diagram did was ask amongst I think it’s fifteen different areas, when the paradigm changes happened in that particular area of society. What he found was that paradigm changes are getting faster and faster. And if you compare our life now to that of my grandmother, when my grandmother was born there were no inside toilets. There was no electricity. My grandfather’s job in his house was to fill up the oil lights in the house, to make sure they were always full. The only what I would call modern inventions that were available when he was born were the steam engine train, and photography. Those were the only modern inventions that existed. Since his time, which was only 120 years ago, we’ve had immensely many new paradigm changes. Television, radio, the car, the aeroplane. It’s incredible.
What this graph shows is that not only are paradigm shifts happening faster, but they’re happening steadily fast at a faster rate. The interesting thing is that this line, you can see it’s a very clear line, crosses the axis sometime in the next hundred years. That is the day, or that is the year, when paradigm changes happen every day. So he’s called it the Singularity because the idea is that is the point when computers become smarter than us and suddenly can start doing things that we can’t think of faster than the rate we could do it. I’m not sure if I will live to see it. Some of you here will live to see it. But it’s going to be a very interesting time.
So closing the loop, it’s interesting the thread of this talk has been that DNA is a sort of memory. True memory evolved. And then language, which allowed you to pass memories. And writing, which records the memories past your death.
Recently, last year, there was a DNA storage breakthrough, where people have discovered how to store digital information in DNA. In fact, 700 terabytes of data in just one tiny gram of DNA. It’s a very slow storage, but it’s a huge storage. In other words, one gram would store the same amount as 14,000 DVDs or a 150kg of hard disks.
So my conclusion is that the reason that humans have succeeded so well in comparison with many other lifeforms is because of the development of language, and therefore the development of memes, which make us much more flexible in our ability to survive because we can do it faster and we can pass it on to more people. Particularly successful memes have caused paradigm shifts in human society, which has generated the next generation of memes. And what we’ve seen is that the rate of change is increasingly exponentially over hundreds of thousands of years. So we’ll be confronted with a very interesting time within the next century, when we may have to contend with six new paradigms at breakfast.
This talk will appear as part of a book later this year. The slides are online, and if you can’t find them you should be considering a new career.
Thank you very much.
Further Reference
This presentation's description and bio for Steven, at the Interaction14 site.
Help Support OpenTranscripts
If you found this useful or interesting, please consider supporting the project at Patreon, or even just sharing the link. It really helps. Thanks.